Basic AI Setup

In order to start setting up more advanced AI, I setup the most base version. I copied the Unreal Third Person Blueprint to re-purpose it for the AI Companion. I removed the camera components and input logic from the AI Companion blueprint. I then added a Pawn Sensing component as shown in the image above.

I added the logic to have the enemy move to the location of the player when the player is sensed visually. The AI’s view is show with the green debug lines.
I then added a Nav Mesh Bounds Volume to the level and enlarged it to encompass the whole level. The result of this most basic AI follow can be seen below.
The major problem with this basic setup is that the AI itself is not very intuitive as it only moves towards the player and doesn’t make any other decisions. This can be solved by using an AI controller, Behavior Trees, and Blackboards. For this project, the core logic will be stored in these blueprints, however, when I get to custom pathfinding, that will be done in C++ using Dijkstra’s Algorithm.
Behavior Trees
Behavior Trees hold the flow logic for the AI. They execute from left-to-right and top-down. For learning purposes, I have followed the Behavior Tree Quick Start Guide in the Unreal documentation. I will be modifying and expanding my BT_Companion to hold all of the logic for the companion AI. This means that some of the logic that currently exists may be removed later. For example, the guide had me add a Patrol sequence, which a Companion AI would probably not need.
Behavior Tree Tasks
Each purple node in the Behavior Tree is a Behavior Tree Task that is specifically used to do an action for a Behavior Tree. In the image above, you can see a custom implementation of a Behavior Tree Task. This implementation from the Quick Start Guide just makes the AI move to random locations for patrolling.
Decorator
The decorator in the behavior tree is just a conditional statement for the behavior tree execution. In the Behavior Tree image, the “Has Line Of Sight?” is the decorator in the “Chase Player” sequence.
Services
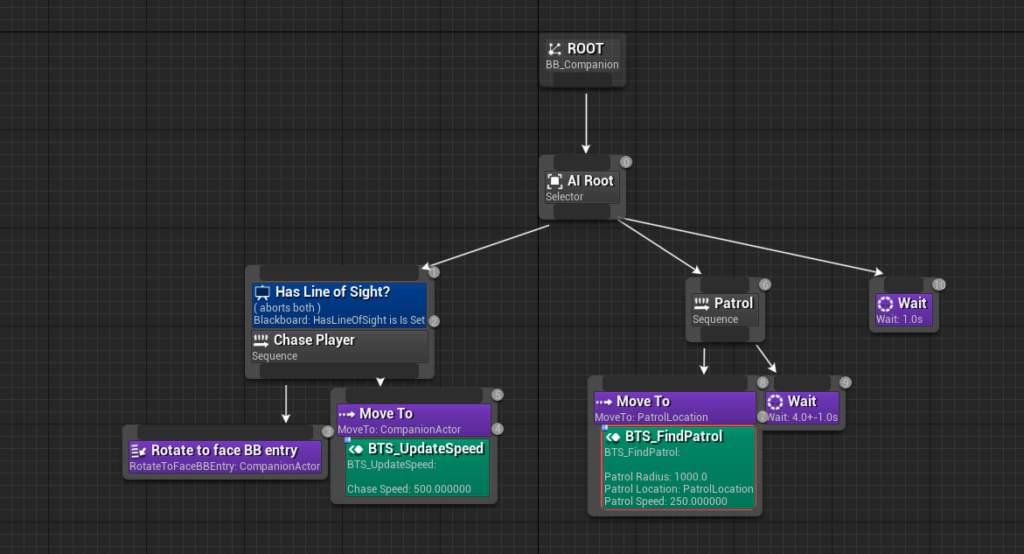
Services can also be added to Behavioral Tree Tasks or Composites. In the above image, I swapped out the tasks for updating speed and finding patrol locations with custom services.
Other types of service include the Default Focus and Run EQS services. The Default Focus service node allows the AI controller to directly access an actor that it is focused on. Then the Run EQS service node executes an Environmental Query System (EQS) template at specified intervals. The EQS collects data from the environment for the use of decision making in Behavior Trees. For example, Actor locations may be collected.
AI Controller
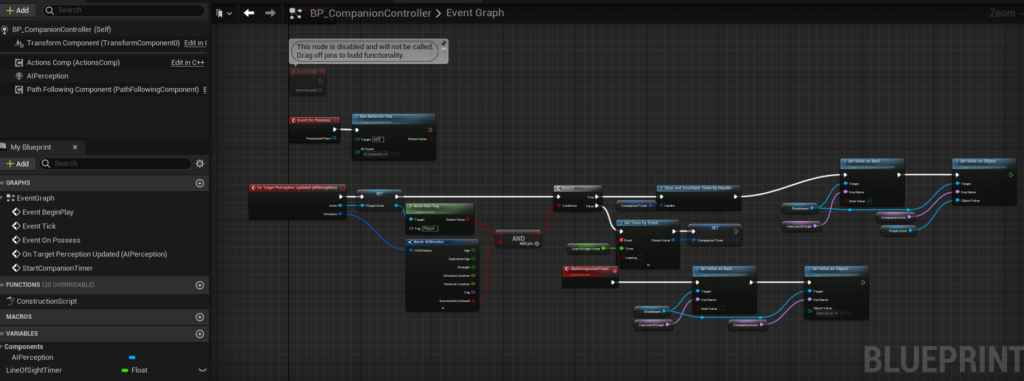
The AI Controller is essentially the root for all decision making for the AI. The primary purpose of the above implementation from the Quick Start Guide is to set the Blackboard values when the player is in its line of sight. It then calls a custom event to reset those values when the player is no longer in the AI’s line of sight after a specified number of seconds.
Blackboard

The Blackboard is the brain of the AI. It holds the values we want the AI to know about and to make decisions based off of. In the image above, the values stored at the specified Blackboard keys are updated inside the AI Controller. When they are updated, then the execution in the Behavior Tree will be updated as well as the state of the Decorators may have changed.
AI Debugging
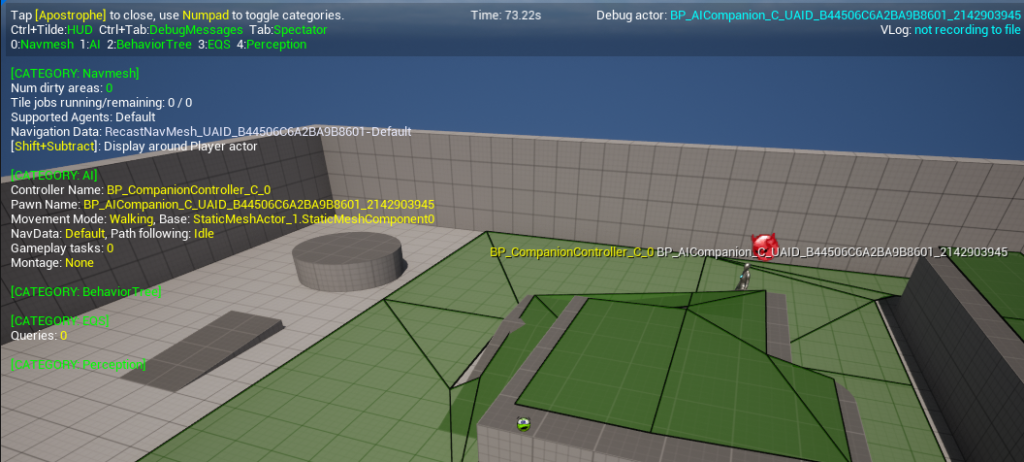
Unreal provides an excellent view for AI Debugging while playing the game. Pressing ‘ (apostrophe) will enable the AI Debugging Tool when running the game. Then you can toggle the different categories with the numpad. This tool will be very valuable in debugging any behavior tree issues that may arise in the future.