First, I’ve decided to deviate from my initial schedule in order to focus on completing the basic functionality of player climbing. This post will walk through my process of enabling the player to climb on and between splines.
Initially, I attempted to implement a system where the player lerps to the first point on the spline and is then able to move along it. During this first attempt, I was setting the player’s location. However, I encountered an issue. After the player initially lerped to the first location, they would eventually drop to the ground and become stuck. This issue is demonstrated in the video below.
First, I attempted to make it so that the player would lerp to the first point on the spline and then be able to move along the spline. In this first attempt, I was setting the player’s location. However, I ran into an issue. When the player initially lerped to the first location, they would eventually drop to the ground and become stuck. This can be seen in the video above.
I tried turning off the wall collision and disabling the player’s gravity, yet the same issue persisted.
Eventually, I fixed the character movement to ensure that after the player correctly lerps to the spline point, they do not fall down. Moreover, the movement along the spline was set to a constant velocity upon key press.
Additionally, the character movement component required all movement to be stopped as soon as the player entered the climbing trigger, ensuring that the original movement input was cleared. Furthermore, to prevent falling, the character movement gravity scale needed to be set to 0.0f.
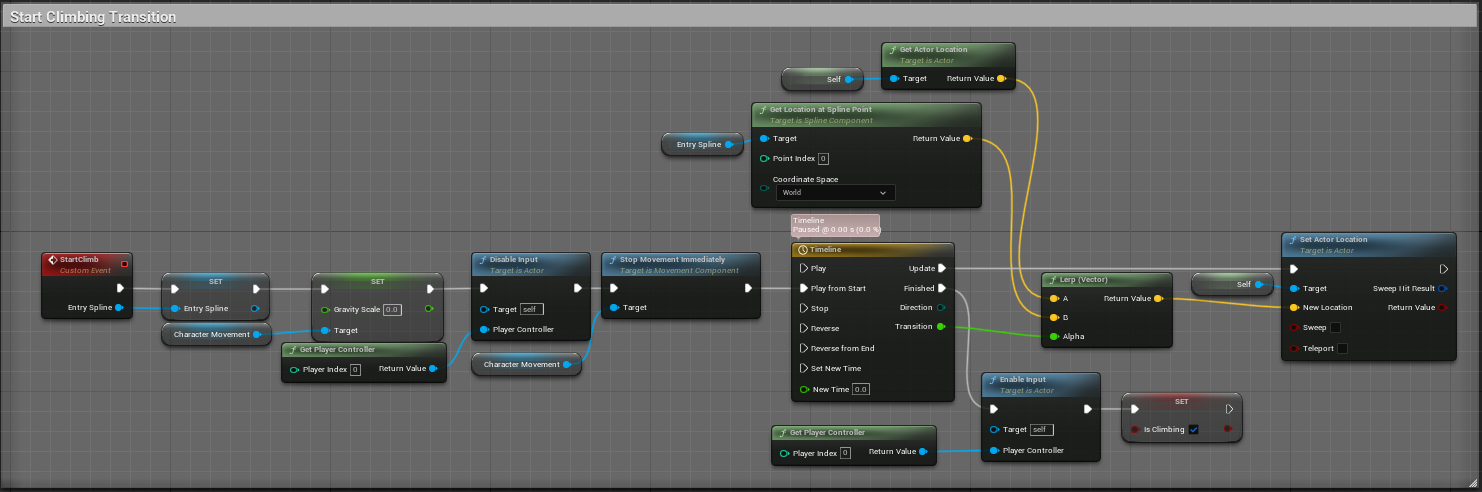
In the above image, you can see the state of the start climb event.
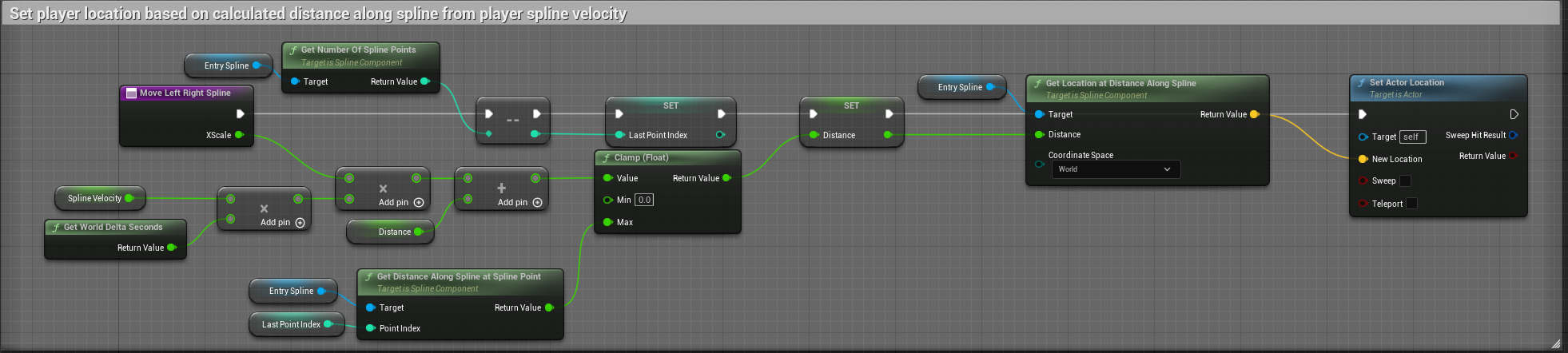
The above image shows how the player’s location was being set for the movement on the spline.
To allow the player to decide when to jump to the next spline, I implemented a functionality where the player needs to press E. The player can only jump to the next spline if they are at the spline point closest to the next spline.
The above example shows the initial implementation of jumping to the next spline. However, at this point, the player was unable to go in reverse order. Additionally, the movement was not smooth due to a bug.
Above is an example of the second pass on jumping to the next spline. Although it still doesn’t support going in reverse, it fixes the issues encountered in the first pass. There were two main issues that needed to be addressed.
Firstly, I realized that I had forgotten to pass the current spline into the “Lerp to Spline” function. This was an oversight as I had previously focused on fixing a different error related to spline validity. Instead, I should have checked what was being passed in initially.
The second issue was related to the player’s movement after jumping to the next spline. Although the jump itself was successful and the player landed at the correct point, pressing right would cause the player to instantly jump to the end. This issue stemmed from not resetting the distance variable, which tracks the player’s position along the spline, to 0 after jumping to the new spline.
Now, the player can successfully transition between both points. The next step is to implement jumping in reverse and enable jumping off the wall.
In the video above, you can observe how the player enters the graph, moves, jumps between splines, and exits the graph. To enter the graph, the player needs to be inside the trigger box while simultaneously pressing E. Subsequently, to jump between the splines, the player must be in close proximity to the spline point and press E to transition to the next spline.
Finally, I made adjustments to the design of the graph system. Initially, I had set up the BP_SplineGraph to have spline components directly attached to them. However, this approach had limitations as it provided minimal control to the designer for adding new splines per graph instance. Furthermore, I was manually adding the splines to an array in the BeginPlay event.
To address these issues, I introduced a separate blueprint called BP_Spline, which contains a spline component. I modified the BP_SplineGraph blueprint to use BP_Spline instances instead of the spline component itself in the array. This change enables designers to easily utilize the picking tool and select specific BP_Spline instances they want to include in the graph directly from the scene. Additionally, they can now rearrange the instances in the array using the details window according to their desired order. The order of the instances in the array determines the path for jumping. It is important to note that this implementation currently only supports linear paths and will require adjustments for more complex graphs.
Furthermore, I incorporated an InstancedStaticMesh into the BP_Spline. This enhancement enables me to set up the ConstructionScript in such a way that it automatically adds static mesh instances to the spline and properly rotates them along the spline.
The next step is to continue expanding the capabilities of the graph system. This includes adding features such as determining which animations should be played, incorporating spline weights for AI, implementing actor filtering, and supporting non-linear pathing.
Blueprints
Interact to next spline point blueprint
Start Climbing Transition
End Climbing Transition
Jump to Next Spline
Move Left and Right on spline
Lerp to Spline
Spline Graph Entry Point and Entering Graph