At the start of this week’s work, I was debugging an issue with the integration of the blueprint setup with the Dijkstra C++ implementation. Every time I ran the pathfinding algorithm, I received the incorrect path. I correctly identified that this was most likely due to the weights from the splines not being updated properly inside the node’s neighbor variable. To fix this, I added the following code inside the AAINode’s “begin play” function. This resolved the primary issue with the code, allowing me to obtain the correct path as seen in the video above. My next step will be to have an AI follow along the precomputed path.
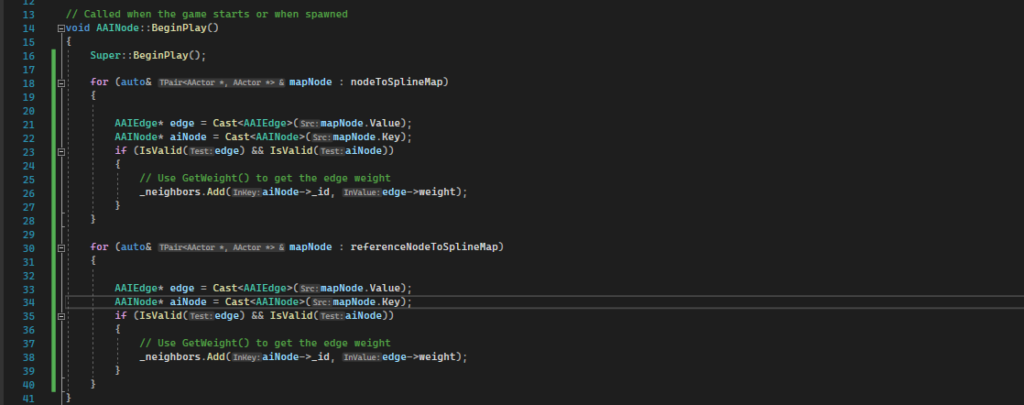
The corrected code can be seen above.
Above you can see the first pass of AI walking along the splines between nodes using the returned path from Dijkstra’s Algorithm. In this video, the AI automatically starts from the start node, but unfortunately is ending one node short. There is a flaw in how I’m getting the AI to traverse to the next node preventing the AI from being able to move to the next node. Additionally, in this implementation, the index for the next node is incremented after the AI has stopped moving. This is less than ideal in a real scenario. It would be better to have it so the AI doesn’t have to stop to progress to the next node. Hopefully, in the future I will be able to refactor this to be better.
In the video above, you can see the AI walking between the nodes in the correct order; however, the movement is not correct. The blueprints are not functioning as intended because the AI should be walking along the spline and not directly to the next node. Additionally, there is a flaw in the logic for determining which node to traverse to when it is in the node to spline map.
I believe the issue revolves around the idea that when a node is in that map, it has already reached the end of the spline. This is because the AI’s location would be at the last point of the spline rather than the first point. On the other hand, the reference node to spline map works the opposite way, where the AI starts from the first point and traverses the distance of the spline. In the video, the AI is strictly walking along only the reference to node splines, but I need it to work from both maps so that the spline’s direction does not matter.
The next steps are to fix the issue with the node to spline map traversal logic and correct the overall spline traversal logic so that the AI actually walks along the spline as intended.

The first fix can be seen above. The blueprints show that I switched the direction on the spline where the AI should lerp across. This fixes the issue where, if the node to spline map was being used for the traversal, the AI wouldn’t traverse properly, as mentioned previously. This means that, to achieve the rest of the primary functionality, I just need to fix the AI not walking along the spline.
Above is an example of the AI walking along the spline. However, the AI behaves oddly when it starts to move towards the next node. Additionally, since I have it incrementing the index for the next node after the AI has stopped, it is prone to mistakes. I will need to come up with a better solution for determining when to increment the index rather than relying on whether the AI is moving.
Part of the solution for getting the AI to walk along the path appropriately was ensuring that the play rate of the timeline reflects more accurately with respect to the character’s maximum walk speed. By using the character’s maximum walk speed and the spline distance to determine the play rate duration, I was able to get more accurate results for traversing along the spline. The next goal is to improve the index incrementing with the hope that it will resolve the remaining issues in the AI’s movement behavior.
The first thing I tried was adding a trigger box to each node and then having it call the event to increment and restart the traversal process whenever the AI entered the trigger box. However, it didn’t seem to improve anything; if anything, it actually made it slightly worse. Therefore, the issue with the AI’s movement is rooted somewhere else.
I also noticed that if I clicked out of the editor, the index would increment when it wasn’t supposed to, causing additional unintended behavior. Additionally, I would prefer the incrementing to be based on the trigger instead. However, I noticed that regardless of whether the index is being updated with the trigger or not, the behavior seems to be unpredictable. As seen in the above video, the AI kind of goes crazy after it reaches the last spline, and even then, the framerate seems to affect the accuracy of the AI’s movement along the spline.
I have been attempting to make this work with blueprints, but it may be simpler to fix if I rewrite the implementation in C++ and expose the function to blueprints.
What’s Next?
First, I will fix the AI movement bug mentioned previously. Lastly, I will update the path based on where the player is located and have the AI follow that path along the spline.